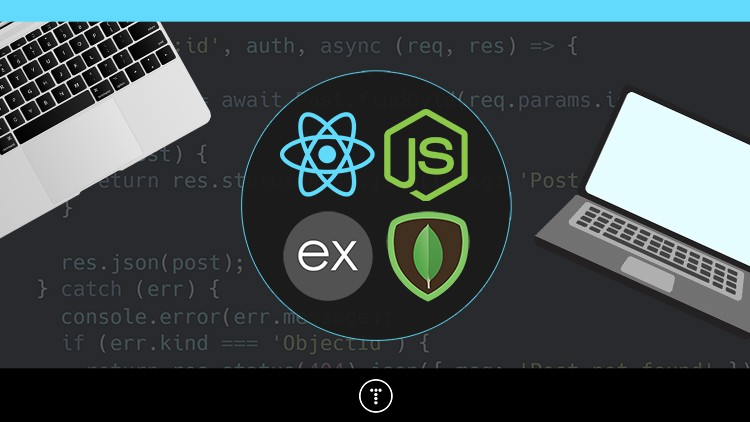
Introduction
Web sockets are a powerful technology for enabling real-time communication in full-stack applications. They allow for persistent, two-way communication channels between a client and a server, which is ideal for scenarios like chat applications, live updates, or real-time notifications. Here is an article that explains how to implement WebSockets in your full-stack application as would be taught in a java full stack developer course.
Understanding WebSockets
WebSockets provide a full-duplex communication channel over a single, long-lived connection. Unlike HTTP, which is a request-response protocol, WebSockets allow the server to push updates to the client at any time, without the client explicitly requesting them.
Implementing WebSockets in a Full-Stack Application
Implementing WebSockets in a full-stack application involves setting up the backend, setting up the front-end, and testing the application. The procedure is explained briefly in this section. It is the practice that you can gain by enrolling in full stack developer classes that will make you proficient in implementing WebSockets in full-stack applications.
1. Setting Up the Backend
You’ll need a server that supports WebSocket communication. Here’s a simple example using Node.js with the ws library:
Install dependencies:
bash
Copy code
npm install ws
Server Code:
javascript
Copy code
const WebSocket = require(‘ws’);
const wss = new WebSocket.Server({ port: 8080 });
wss.on(‘connection’, ws => {
console.log(‘New client connected’);
// Send a message to the client
ws.send(‘Welcome to the WebSocket server!’);
// Listen for messages from the client
ws.on(‘message’, message => {
console.log(`Received: ${message}`);
// Broadcast message to all clients
wss.clients.forEach(client => {
if (client.readyState === WebSocket.OPEN) {
client.send(message);
}
});
});
ws.on(‘close’, () => {
console.log(‘Client disconnected’);
});
});
console.log(‘WebSocket server is running on ws://localhost:8080’);
2. Setting Up the Frontend
On the frontend, you can use the native WebSocket API provided by the browser.
Client Code:
javascript
Copy code
const ws = new WebSocket(‘ws://localhost:8080’);
// Connection opened
ws.addEventListener(‘open’, () => {
console.log(‘Connected to WebSocket server’);
// Send a message to the server
ws.send(‘Hello Server!’);
});
// Listen for messages
ws.addEventListener(‘message’, event => {
console.log(‘Message from server:’, event.data);
// You can update the UI with the received message
document.getElementById(‘output’).textContent += event.data + ‘\n’;
});
// Handle connection close
ws.addEventListener(‘close’, () => {
console.log(‘Disconnected from WebSocket server’);
});
// Handle errors
ws.addEventListener(‘error’, error => {
console.error(‘WebSocket error:’, error);
});
HTML for Displaying Messages:
html
Copy code
<div id=”output”></div>
3. Testing the Application
- Start the server:
Run your Node.js server with the command:
bash
Copy code
node server.js
- Open the client:
Create a simple HTML file containing the client-side JavaScript, and open it in your browser. The WebSocket connection should be established, and you should see messages being exchanged between the client and server.
Handling Advanced Use Cases
For more complex applications, you might need to:
- Authenticate WebSocket connections: Pass authentication tokens during the connection handshake.
- Scale WebSocket servers: Use tools like Redis for pub/sub messaging across multiple server instances.
- Handle reconnections: Implement logic to reconnect the WebSocket if the connection drops.
Enroll in an advanced java full stack developer course to learn how to implement WebSockets in complex full-stack applications.
WebSocket Libraries and Frameworks
- Socket.IO: Provides a more feature-rich solution, including fallbacks for older browsers that don’t support WebSockets.
- SockJS: Aims to emulate WebSockets and provides automatic fallbacks.
- SignalR (for .NET): A library for ASP.NET developers for real-time communication.
Security Considerations
Most full stack developer classes will include elaborate sessions on the security concerns involved in implementing WebSockets in full-stack applications.
- Encryption: Always use wss:// (WebSocket Secure) in production to encrypt data in transit.
- Authentication: Ensure only authenticated users can establish WebSocket connections.
- Rate Limiting: Implement rate limiting to prevent abuse and DoS attacks.
Conclusion
WebSockets are a versatile and efficient way to add real-time communication features to your full-stack app. By setting up a WebSocket server on the backend and connecting to it from the frontend, you can create interactive, real-time experiences for your users. Full-stack developers can gain the technical know-how and the skills for this by enrolling in an advanced java full stack developer course.
Business Name: ExcelR – Full Stack Developer And Business Analyst Course in Bangalore
Address: 10, 3rd floor, Safeway Plaza, 27th Main Rd, Old Madiwala, Jay Bheema Nagar, 1st Stage, BTM 1st Stage, Bengaluru, Karnataka 560068
Phone: 7353006061
Business Email: [email protected]